Critical Movies
An app that shows New York Times movie reviews with a focus on Critic’s Picks.
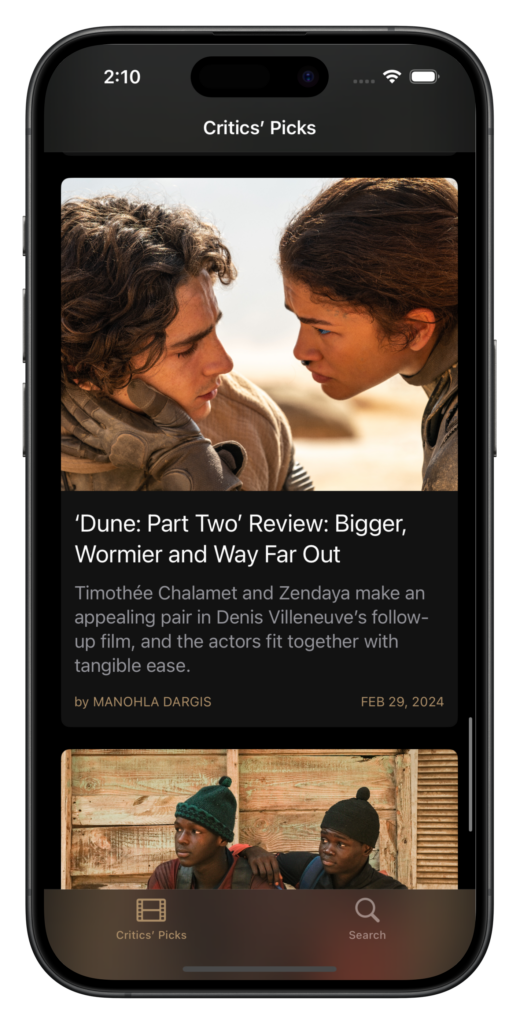
Why I Made This
A UIKit Project
The motivation for this app was driven by a few intersecting things. I wanted to make something with UIKit. I wanted to explore modern collection views, diffable data source and compositional layouts. I thought something content-based would be a good fit and I love watching movies.
My Approach
Programmatic Views and Auto Layout
The primary navigation for this app is a tab bar. One tab shows recent New York Times movie reviews that were a Critic’s Pick. The other enables a search of all New York Times movie reviews and results indicate which movies were a Critic’s Pick.
I chose to write views and Auto Layout in code. I like the explicitness of writing everything out but there are well-known trade-offs.
Features
New York Times Movie Reviews
Critic’s Picks movies in reverse chronological order. Tapping a movie opens the full review in a Web view.
Search
The second tab enables users to search all New York Times movie reviews and results that were awarded a Critic’s Pick are labelled.
Infinite Scroll
Both lists feature infinite scrolling that loads more results from the network until it indicates there are no more results.
Frameworks
UIKit
The app has a UITabViewController
at its root, a view controller at the base of each tab and a single, reused collection view for movie reviews.
UICollectionView
Each collection view implementation uses a diffable data source and compositional layout, and injects a different cell for movie reviews.
Auto Layout
Both cells adapt their height based on varying movie title and description lengths. The search cell conditionally adds a Critic’s Pick label.
XCTest
Unit-tested view controllers, search controller, collection view and movie service using techniques from Jon Reid’s iOS Unit Testing by Example.
URLSession
Separate objects fetch data and images. The image service tracks requests to implement onReuse based on Donny Wals’s approach.
Codable
Model objects mimic the JSON hierarchy returned by the New York Times API and properties are renamed using CodingKeys.
Architecture & Design Patterns
Organizing, Reorganizing and Reusing Code
I figured out the content and functionality of the app as I went along and the best way to organize the code changed as the app grew.
- I initially decided the loading view and content loading behavior belonged with the collection view, to be reused as one whole thing. However, when I reused the collection view, I needed the loading view to appear and behave differently. I separated the loading behavior into its own view controller and made the collection view controller inherit it.
- In order to make the collection view reusable, I needed the ability to use it with different cells and compositional layouts. I created a MovieCell protocol to ensure cells were able to populate movie data and request movie images. Both the cell and compositional layout are injected when the collection view is initialized.
- I split the movie service object into separate data and image services. Only the image service was using the cache and needed to keep track of network requests in order to implement onReuse in the collection view.
- Containing view controllers act as delegates for their collection views to load movies and full New York Times reviews in a Web view. The search tab view controller implements
UISearchBarDelegate
methods as well.
Up Next
Better UX, More Layouts
There’s a lot left to do. I’ve outlined some of the things I plan to add or fix below:
- The search tab needs a better experience with placeholder searches and error handling that accounts for all possible search and result states.
- I want to experiment with additional compositional layouts for larger screen sizes. It will involve thinking about the Auto Layout constraints of each cell as well as the compositional layout since they work in tandem.